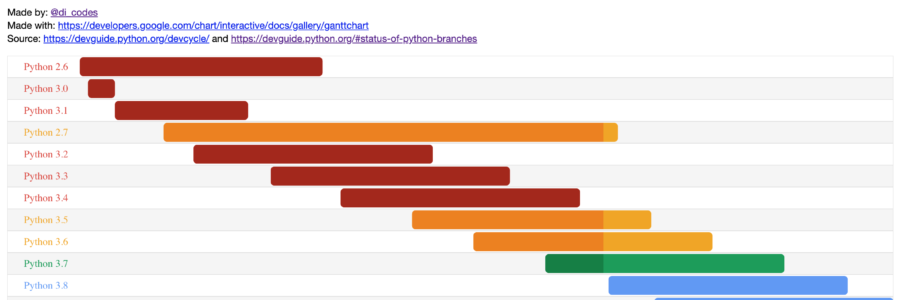
Python versions
Overview of major enhancements in each version
Python 3.6 (released December 2016)
Formatted string literals (f-strings):
name = "World" print(f"Hello, {name}!")
Underscores in Numeric Literals:
billion = 1_000_000_000
Variable Annotations:
age: int = 25
Python 3.7 (released June 2018)
Data Classes:
from dataclasses import dataclass @dataclass class Point: x: float y: float
Asyncio Enhancements:
async def main(): await asyncio.sleep(1) print('hello')
Built-in breakpoint():
breakpoint() # Enters the debugger here.
Python 3.8 (released October 2019)
Walrus Operator (:=): if (n := len(a)) > 10: print(f"List is too long ({n} elements)")
Positional-Only Parameters:
def func(a, b, /, c, d): return a + b + c + d
Python 3.9 (released October 2020)
Dictionary Merge (|) and Update (|=) Operators:
x = {"key1": "value1"} y = {"key2": "value2"} z = x | y
Type Hinting Generics in Standard Collections:
def greet_all(names: list[str]): for name in names: print("Hello", name)
Python 3.10 (released October 2021)
Structural Pattern Matching:
def http_error(status): match status: case 404: return "Not found" case 401 | 403: return "Not allowed"
Parenthesized Context Managers:
with (open('file1.txt') as f1, open('file2.txt') as f2): data = f1.read() + f2.read()
Python 3.11 (released October 2022)
Faster CPython: Performance improvements.
Exception Groups and except*:
try: raise ExceptionGroup("Errors", [ValueError("wrong value"), TypeError("wrong type")]) except* ValueError as e: print("Handled ValueError:", e)
Python 3.12 (released in October 2023)
Function Overloading with PEP 690:
class Calculator: @overload def add(self, a: int, b: int) -> int: ... @overload def add(self, a: float, b: float) -> float: ... def add(self, a, b): return a + b