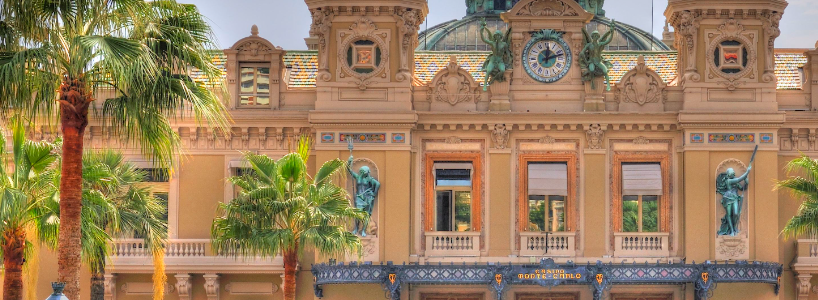
Roll the dice! Monte Carlo Simulations in Python
Monte Carlo simulations are a powerful tool used to model a wide range of phenomena in finance, engineering, physics, and other fields. They are based on the concept of random sampling and are particularly useful for situations where the underlying system is too complex to model using traditional mathematical techniques.
In this blog post, we will explore Monte Carlo simulations using Python, a popular and versatile programming language used in data analysis, machine learning, and scientific computing.
What is Monte Carlo Simulation?
Monte Carlo simulation is a technique that uses random sampling to model complex systems. It is named after the Monte Carlo Casino in Monaco, where games of chance are based on random outcomes. In a Monte Carlo simulation, we use random numbers to simulate a system repeatedly, and we observe the behavior of the system based on these simulations.
The basic steps of Monte Carlo simulation are:
- Define the system to be simulated
- Identify the input parameters that affect the system
- Define a range of possible values for each input parameter
- Generate a set of random values for each input parameter
- Run simulations for each set of input values
- Analyze the results of the simulations
Monte Carlo simulations are particularly useful for modeling systems that are subject to random variation or uncertainty. For example, financial models often use Monte Carlo simulations to model stock prices, interest rates, and other variables that are subject to fluctuations.
Monte Carlo simulations can also be used to solve complex problems, such as optimization problems or integrals, that cannot be solved using traditional mathematical techniques.
Monte Carlo Simulation in Python
Python is a popular language for Monte Carlo simulations because it is easy to use, has a wide range of libraries, and is efficient for numerical computations. In this section, we will walk through an example of a Monte Carlo simulation using Python.
Example: Rolling Dice
Suppose we want to simulate rolling two dice and calculate the probability of rolling a sum of 7. We can use a Monte Carlo simulation to approximate the probability of rolling a 7 by generating a large number of random rolls and counting the number of times the sum is 7.
Here’s the Python code for this simulation:
import random
# Define number of rolls
n = 1000000
# Count the number of times we roll a 7
count = 0
# Simulate rolling two dice n times
for i in range(n):
roll1 = random.randint(1, 6)
roll2 = random.randint(1, 6)
if roll1 + roll2 == 7:
count += 1
# Calculate the probability of rolling a 7
p = count / n
print("Probability of rolling a 7:", p)
In this code, we first import the random
library, which contains functions for generating random numbers. We then define the number of rolls n
and set the initial count to zero.
We use a for
loop to simulate rolling two dice n
times. In each iteration, we generate random numbers between 1 and 6 to simulate rolling the two dice. If the sum of the two rolls is 7, we increment the count.
Finally, we calculate the probability of rolling a 7 by dividing the count by the total number of rolls n
.
When we run this code, we get the following output:
Probability of rolling a 7: 0.166615
This indicates that the probability of rolling a 7 is approximately 1/6, which is what we would expect based on the theory of probability.
Conclusion
Monte Carlo simulations are a valuable technique for modeling complex systems and solving difficult problems. They rely on random sampling to simulate a system repeatedly, allowing us to observe its behavior and analyze the results.
Overall, Monte Carlo simulations using Python are a valuable tool for any data analyst, scientist, or engineer looking to model complex systems and solve difficult problems