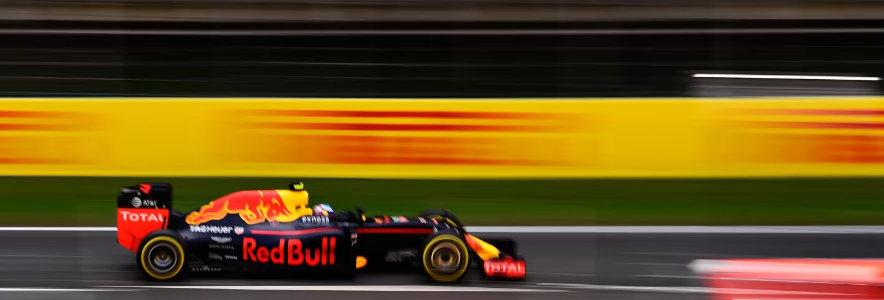
High Performance Python
Python is a high-level programming language known for its ease of use and readability. However, its interpreted nature often leads to performance issues when compared to compiled languages like C and C++. In this blog post, we’ll explore some tips and techniques that can be used to achieve high performance in Python and compare them with their counterparts in C and C++.
Use NumPy and pandas for data manipulation
NumPy and pandas are Python libraries that offer high-level data manipulation capabilities. These libraries are built on top of highly optimized C and Fortran libraries, which means that they are faster than pure Python alternatives. When working with large datasets, it’s recommended to use NumPy and pandas to achieve better performance.
In C and C++, similar functionalities can be achieved by using built-in data types and libraries like the Standard Template Library (STL) or Boost. However, these require more low-level coding and understanding of memory management.
Use Cython to speed up Python code
Cython is a superset of Python that allows you to write C extensions for Python. Cython is essentially a hybrid language that allows you to write Python code with C-like syntax. Cython can be used to speed up Python code by compiling it to C, which can then be compiled to machine code. This results in a significant performance boost.
In C and C++, code can be written natively, which means that there is no need for a separate compiler. However, Cython can be used to write Python-like code that is then compiled to C or C++, which can result in significant performance improvements.
Use multiprocessing for parallel processing
Python’s Global Interpreter Lock (GIL) is a mechanism that ensures only one thread can execute Python bytecode at a time. This means that when running CPU-bound tasks, Python’s performance is limited to a single core. Multiprocessing is a Python library that can be used to overcome this limitation by allowing multiple processes to execute Python code in parallel.
In C and C++, multiprocessing can be achieved using low-level threading libraries like POSIX threads (pthread) or Windows threads. These require more complex coding and understanding of thread synchronization.
Use JIT compilers
Just-In-Time (JIT) compilers can be used to optimize Python code at runtime. JIT compilers work by analyzing the code that is being executed and generating optimized machine code. This can result in significant performance improvements.
In C and C++, code is compiled ahead of time, which means that it is optimized before runtime. However, JIT compilers can also be used in C and C++, for example, the LLVM JIT compiler.
Avoid using global variables
Python’s performance can be negatively impacted by the use of global variables. This is because accessing global variables requires the interpreter to perform a dictionary lookup, which can be slow. To achieve better performance, it’s recommended to use local variables or class attributes instead of global variables.
In C and C++, global variables are also discouraged as they can lead to memory management issues, especially in large-scale programs.
Calling C/C++ methods from Python
To call a C or C++ method from Python, you can use the ctypes library, which allows you to create Python bindings for C functions.
Here is an example of how to call a C function from Python using ctypes:
- First, create a C file with the function you want to call. For example, let’s say you have a file called “example.c” with the following function:
// example.c
int add(int a, int b) {
return a + b;
}
- Next, compile the C file into a shared library using a C compiler. For example, if you’re using GCC on Linux, you can run the following command:
gcc -shared -o example.so example.c
This will create a shared library called “example.so” that contains the add function.
- In Python, import the ctypes library and load the shared library using the
CDLL
function:
import ctypes
lib = ctypes.CDLL('./example.so')
Note that you may need to provide the full path to the shared library if it’s not in the current directory.
- Finally, you can call the C function from Python using the
lib
object and passing the function name and arguments:
result = lib.add(3, 4)
print(result) # Output: 7
This will call the add
function from the shared library and return the result, which in this case is 7.
You can also specify the return type and argument types using the restype
and argtypes
attributes of the function object, respectively.
# Specify return type and argument types
lib.add.restype = ctypes.c_int
lib.add.argtypes = [ctypes.c_int, ctypes.c_int]
# Call the function
result = lib.add(3, 4)
print(result) # Output: 7
Conclusion:
In summary, achieving high performance in Python requires a combination of optimizing code, using external libraries, and taking advantage of the available tools like JIT compilers and multiprocessing. While C and C++ offer better performance out of the box, Python’s ease of use and high-level abstractions make it a popular choice for many developers. However, it’s important to keep in mind that performance is a tradeoff between simplicity and speed, and the choice of language should be made based on the specific requirements of the project.