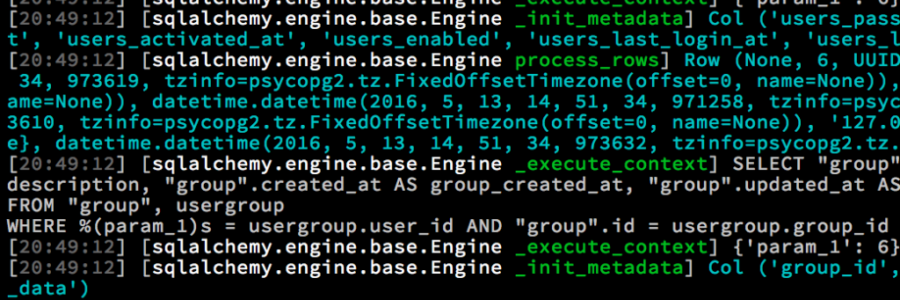
Setting up Python logging efficiently
The Python logging framework has been around for a long time, and with such a mature framework, it’s quite natural that the number of features and extensions have grown over the years.
To some extent, I would say that the “logging” package almost is a case of “featuritis”. It is VERY capable, and configurable almost to a degree that is incomprehensible to a lowly createure like myself.
Therefore, this short article.
Use Cases
99 times of 100, you have one or the other of the following use cases:
- You want to print to screen only, but with line numbers etc to ease debugging
- You want to print to screen, and also log to a file
This is how you do it:
import logging fileHandler = logging.FileHandler("datasender.log") consoleHandler = logging.StreamHandler() logging.basicConfig(level=logging.INFO, datefmt='%Y-%m-%d %H:%M:%S', handlers=[fileHandler, consoleHandler], format="%(asctime)s [%(name)-12.12s] [%(levelname)-5.5s] %(message)s")
The key is of course here to create the Handlers first, then pass an array of them to the basicConfig. That way you get all the other arguments the same for all handlers. Pretty neat, I think.
Then in your code, whenever you need a logger you do:
logger = logging.getLogger("DataSender")
where the argument will be used as $(name) in the template. So if you want to keep parts of the application apart you can just get a logger with that name.
This is my preferred way od doing it now.