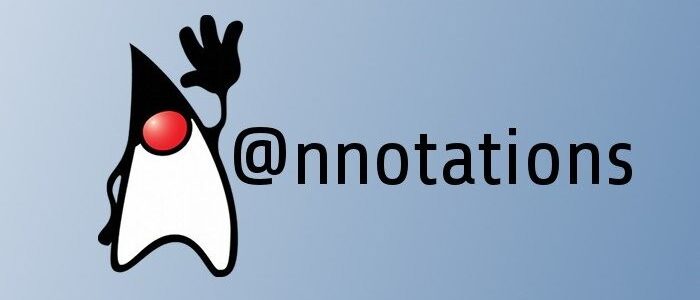
Using annotated Spring Boot entities in a Library
When I moved a bunch of classes from a rather large Spring Boot application into a separate library (se more about that process here), I immediately got the problem that the annotated classes weren’t found from my application.
This happens because the packages aren’t automatically scanned, so Spring doesnt know about them.
The solution turned out to be quite simple. I found it on StackOverflow of course: https://stackoverflow.com/questions/41815871/spring-boot-autowire-beans-from-library-project
Add a configuration class like this:
package com.myProject.customLibrary.configuration;
@Configuration
@ComponentScan("com.myProject.customLibrary.configuration")
@EnableJpaRepositories("com.myProject.customLibrary.configuration.repository")
@EntityScan("com.myProject.customLibrary.configuration.domain")
public class SharedConfigurationReference {}
Then in your application use:
@SpringBootApplication
@Import(SharedConfigurationReference.class)
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
The annotated beans are now accessible from your application.
Note the comment:
“However, if you want to avoid importing the configuration class. You can create a folder called ‘META-INF’ in the ‘resources’ folder of your library and add a file called ‘spring.factories’ with the content org.springframework.boot.autoconfigure.EnableAutoConfiguration=<fully_qualified_name_of_configuration_file>
. This will autoconfigure your library.”
This sounds promising, however I haven’t tried that approach.