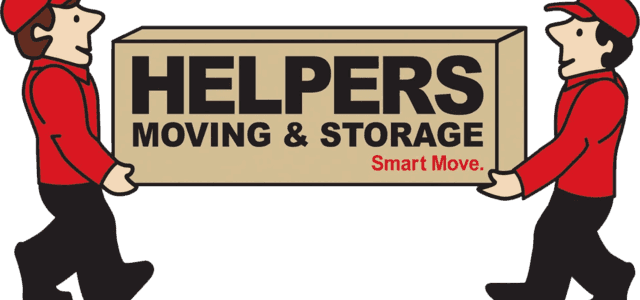
Make your own Rails View helpers
Rails helpers like image_url, link_to, form_with, fields_for etc are nifty little “macros” that makes your Rails code smaller and clearer.
I mean, who doesn’t prefer
link_to "Click here",categories_path, :class=>'myclass'
to
<a class="myclass" href="/categories">Click here</a>
or even worse, when inserting Ruby into HTML
<a class="myclass" href=<%= categories_path %> >Click here</a>
Helper makes your code easier to read, and more Ruby-esque!
Example
I am using bootstrap, and I need a number of input fields like these:
<div class="input-group mb-3"> <div class="input-group-prepend"> <span class="input-group-text">Category</span> </div> <input type="text" class="form-control" placeholder="Category"> </div>
A perfect candidate for a helper
I could create a GEM with a Module for this, but in this example I choose to put the helper in application_helper.rb
module ApplicationHelper def bs4_input(name) content_tag :div, class: "input-group mb-3" do concat(input_group(name.to_s)) concat(form_control(name.to_s)) end end def input_group(name) content_tag :span, :class => "input-group-text" do name end end def form_control(name) content_tag :input, class:"form-control", :placeholder => name do end end end
Note that this is a simplified example, in the real world I would add more options like id, class etc. Normally you pass these in an options {} hash. But now you can use the helper like this
<%= bs4_input :title%> <%= bs4_input :category%>
Nice, isnt it?