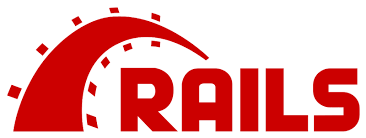
Redefining Rails Models: Going Beyond Rails and Sometimes, Beyond Tables
Harnessing ActiveRecord Outside Rails
ActiveRecord, the model component of Rails, is well-known for its versatility. It can be used within Rails, of course, but did you know it can also be used in a standalone Ruby application? Yes, indeed!
To utilize ActiveRecord in a vanilla Ruby setting, you’ll need to add the ‘active_record’ gem to your Gemfile or require it in your script as shown below:
require 'active_record'
However, unlike when using Rails, ActiveRecord won’t automatically connect to your database. You’ll need to establish the connection manually. Don’t worry, it’s as simple as this:
# Substitute the following values based on your database settings
ActiveRecord::Base.establish_connection(
adapter: 'mysql2', # Or 'postgresql' or 'sqlite3' or 'oracle_enhanced'
host: 'localhost',
database: 'your_database',
username: 'your_username',
password: 'your_password'
)
Once connected, you can easily interact with your database tables. To achieve this, simply inherit a class from ActiveRecord::Base:
# Assume the table name is "products"
class Product < ActiveRecord::Base
end
But what if the table name is unconventional? No problem, ActiveRecord is prepared for such situations. If you’re using Rails 3.2 or older, use set_table_name
, but for newer Rails versions, use self.table_name
:
rubyCopy code# BEFORE Rails 3.2
set_table_name "unconventional_products"
# AFTER Rails 3.2
self.table_name = "unconventional_products"
Models sans Tables: A Possibility Worth Exploring
ActiveRecord provides a lot of fantastic features. But sometimes – or, let’s be honest, quite often – we find ourselves wanting a model with all the perks of ActiveRecord without being tied to a database table.
Introducing ActiveModel::Model
! This module includes methods for model naming, conversions, translations, and validations, which can be handy for various cases. For example:
class ContactForm
include ActiveModel::Model
attr_accessor :name, :email, :message
validates :name, :email, :message, presence: true
end
Here, ContactForm
behaves like an ActiveRecord model, complete with accessors and validations, but it isn’t backed by a database table.
Desiring a more “Rails-like” environment, complete with RAKE but without Rails itself? That can also be achieved! Check out this detailed guide: https://gist.github.com/schickling/6762581.
So, with a bit of ingenuity and flexibility, we can experience the benefits of Rails, ActiveRecord, and RAKE – all without being confined to Rails or even a database table!