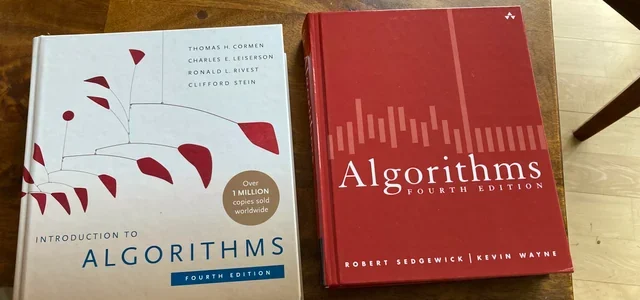
The Major Algorithms You Should Study to Solve AdventOfCode Problems
If you’ve ever participated in AdventOfCode, you’ll know it’s a thrilling yet challenging journey of solving programming puzzles. While your language of choice matters, the key to success often lies in understanding the algorithms and techniques that underpin efficient solutions. Here’s a curated list of the major algorithms you should study to tackle AdventOfCode problems like a pro.
1. Pathfinding and Graph Algorithms
Many AdventOfCode problems revolve around grids, mazes, or connected systems, making graph algorithms essential.
- Breadth-First Search (BFS): Ideal for finding the shortest path in unweighted graphs.
- Example: Traverse a maze to find the shortest route.
- Depth-First Search (DFS): Useful for exploring all possible paths or solving recursive problems.
- Example: Detect cycles or find connected components.
- Dijkstra’s Algorithm: Solves shortest-path problems in weighted graphs efficiently.
- Example: Calculate the least costly route between points.
- A* Search Algorithm**: A more focused pathfinding algorithm using heuristics.
- Example: Navigate a grid with weighted nodes efficiently.
- Topological Sorting: Useful for problems involving dependency resolution.
- Example: Determine task execution order.
2. Dynamic Programming (DP)
Dynamic Programming is crucial for solving optimization problems by breaking them into subproblems.
- Knapsack Variants: Learn the 0/1 Knapsack problem to handle constrained optimization.
- Example: Select items to maximize value without exceeding weight.
- Longest Common Subsequence (LCS): A foundation for string comparison problems.
- Example: Find similarities between sequences.
- Memoization and Tabulation: Understand these techniques to optimize recursive calls.
- Example: Avoid recomputing values in overlapping subproblems.
- Subset Sum and Partition Problems: Useful for problems involving subsets of integers.
- Example: Determine if a set can be split into two equal-sum subsets.
3. Greedy Algorithms
Some problems can be solved by making locally optimal choices that lead to a global solution.
- Activity Selection: Maximizes the number of non-overlapping intervals.
- Example: Schedule tasks to minimize idle time.
- Huffman Encoding: Learn this for compression and frequency-based encoding problems.
- Example: Create an optimal encoding for characters.
- Minimum Spanning Tree (Prim’s/Kruskal’s): Solve problems involving connecting points with minimal cost.
- Example: Design a cost-efficient network.
4. String Algorithms
String manipulation and pattern matching are common in AdventOfCode.
- Knuth-Morris-Pratt (KMP): Efficiently searches for substrings.
- Example: Find patterns in large texts.
- Z-Algorithm: Another powerful string matching algorithm.
- Example: Identify all occurrences of a pattern.
- Suffix Arrays and Trees: Solve problems related to substring queries.
- Example: Find the longest repeated substring.
- Edit Distance (Levenshtein): Useful for problems involving transformations.
- Example: Calculate the minimum steps to convert one string to another.
5. Computational Geometry
Occasionally, AdventOfCode will involve spatial data or geometry.
- Convex Hull: Determine the smallest convex boundary around a set of points.
- Example: Identify boundaries of points in a 2D space.
- Line Intersection: Check if two lines intersect or calculate the intersection point.
- Example: Solve puzzles involving overlapping paths.
- Closest Pair of Points: Efficiently find the nearest neighbors in a set of points.
- Example: Determine proximity in a grid or map.
6. Divide and Conquer
Divide and conquer algorithms break problems into smaller subproblems and combine solutions.
- Merge Sort and Quick Sort: Efficient sorting techniques.
- Example: Sort large datasets in O(n log n) time.
- Binary Search: Find elements efficiently in sorted data.
- Example: Locate a value or satisfy a condition within constraints.
- Fast Exponentiation: Solve problems involving modular arithmetic quickly.
- Example: Compute large powers mod n efficiently.
7. Backtracking and Constraint Satisfaction
When brute force is required but can be optimized, backtracking shines.
- N-Queens Problem: Place queens on a chessboard without conflicts.
- Example: Solve grid-based placement problems.
- Sudoku Solver: Learn to fill constraints in grids recursively.
- Example: Solve puzzles with specific rules.
- Hamiltonian Paths and Cycles: Navigate through graphs visiting nodes exactly once.
- Example: Solve pathfinding problems with strict constraints.
8. Data Structures for Efficient Computation
The right data structure can dramatically simplify problems.
- Priority Queues and Heaps: Solve problems involving dynamic ordering.
- Example: Implement Dijkstra’s algorithm.
- Disjoint Set Union (Union-Find): Handle connected components efficiently.
- Example: Solve problems involving merging sets.
- Segment and Fenwick Trees: Useful for range queries and updates.
- Example: Aggregate data across intervals.
- Tries: Efficiently store and query strings or prefixes.
- Example: Solve prefix-based search problems.
9. Specialized Algorithms
Some problems require more advanced or niche techniques.
- Fast Fourier Transform (FFT): Useful for polynomial multiplication and signal processing.
- Example: Solve numerical problems involving cyclic patterns.
- Monte Carlo and Randomized Algorithms: Approximation methods for uncertain or probabilistic scenarios.
- Example: Simulate outcomes in complex systems.
- Bit Manipulation: Solve problems involving binary operations efficiently.
- Example: Find subsets, XOR operations, or count bits.
Conclusion
AdventOfCode problems are diverse and span a wide range of algorithmic challenges. By focusing on the algorithms listed above, you’ll be well-equipped to tackle most puzzles efficiently. Start by mastering the fundamentals like graph traversal, dynamic programming, and sorting algorithms, and gradually expand to specialized techniques as needed.
Happy coding and may your solutions be both correct and efficient!