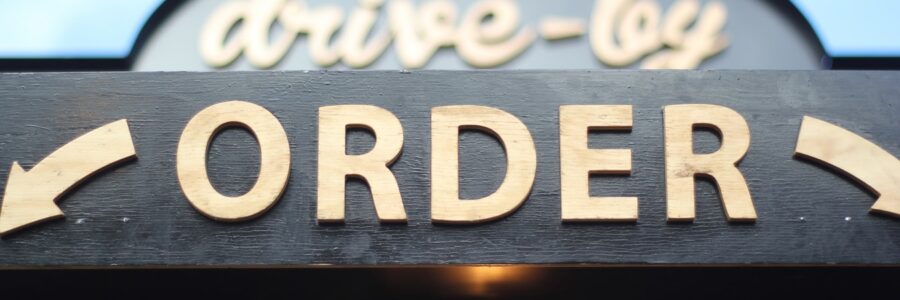
Tracking Orders with Interactive Brokers API: The Importance of reqOpenOrders and Client ID
When working with the Interactive Brokers (IB) API, one of the most essential components for effective order tracking is understanding the role of client ID and using reqOpenOrders()
to ensure accurate tracking of orders made by your application. In this post, we will focus primarily on using reqOpenOrders()
, the most common and reliable way to track orders, emphasizing how the client ID plays a vital role in differentiating and managing orders. Later, we will cover other methods like reqAllOpenOrders()
and reqAutoOpenOrders()
as special use cases.
Tracking Orders with reqOpenOrders()
and the Role of Client ID
The reqOpenOrders()
method is the core approach for tracking open orders that are placed by a specific client connection to TWS or the IB Gateway. It works effectively by isolating the orders tied to a specific client ID, providing clear, distinct information about orders originating from that particular session or application instance.
Why is Client ID Important?
- Client ID is a unique identifier assigned to each API connection to the TWS or IB Gateway. When using the IB API, each client ID is treated independently. This means:
- Orders placed with one client ID will only be visible to that same client ID when using
reqOpenOrders()
. - Orders placed via manual entry in TWS or via a different client ID will not be visible to the current client connection when you use
reqOpenOrders()
.
How to Use reqOpenOrders()
Effectively
- Assign Unique Client IDs: Each instance of your application should connect to TWS using a unique client ID. This allows you to keep your orders separate from those placed by other applications or users.
- Use
reqOpenOrders()
to Track Orders: This function is straightforward—it requests all open orders placed by the client connection using the specified client ID. - Callback Handling: When
reqOpenOrders()
is called, theopenOrder()
callback will be triggered for each open order. This allows you to update your internal tracking system with the details of the order. - Best for Automated Strategies: If you are running a single automated trading strategy,
reqOpenOrders()
is the most reliable way to ensure that you are only working with orders that your strategy has placed.
Example: Using reqOpenOrders()
for Order Tracking
Here’s a basic example of how you can use reqOpenOrders()
in your application to retrieve open orders placed by your client ID:
from ibapi.client import EClient
from ibapi.wrapper import EWrapper
class IBApp(EWrapper, EClient):
def __init__(self, client_id):
EClient.__init__(self, self)
self.client_id = client_id
def nextValidId(self, orderId):
# Request open orders when the connection is established
self.reqOpenOrders()
def openOrder(self, orderId, contract, order, orderState):
print(f"OpenOrder. ID: {orderId}, Contract: {contract.symbol}, Action: {order.action}, Quantity: {order.totalQuantity}")
if __name__ == "__main__":
app = IBApp(client_id=1001) # Example client ID
app.connect("127.0.0.1", 7496, app.client_id)
app.run()
- In this example, the client ID is set to
1001
, andreqOpenOrders()
is called once the connection is established to retrieve orders related to this specific client.
Special Cases: reqAllOpenOrders()
and reqAutoOpenOrders()
While reqOpenOrders()
is the standard method for tracking orders placed by your client, there are special cases where you might need to use reqAllOpenOrders()
or reqAutoOpenOrders(True)
.
1. reqAllOpenOrders()
- What It Does: Requests a one-time snapshot of all open orders across all clients and manual entries in TWS.
- When to Use: If you need a complete overview of all orders, regardless of which client placed them,
reqAllOpenOrders()
is useful. - Limitation: This is a one-time request—it does not provide continuous updates. To stay up to date, you need to call it periodically.
- Typical Use Case: This is commonly used when multiple systems or manual interventions are involved, and you want to consolidate a complete snapshot.
2. reqAutoOpenOrders(True)
- What It Does: Subscribes to continuous updates for all open orders, similar to a live feed. It provides updates on both manually placed orders and API orders.
- Client ID Requirement: This method only works when using
clientId=0
, also known as the MASTER client. - Potential Issues: It can be unreliable for consistently catching all manual orders due to TWS settings or connectivity stability. Therefore, it may not always be sufficient for accurate tracking of manually placed orders.
- Typical Use Case: It’s useful when you need real-time updates on all order activities, but it should be supplemented with other tracking methods to ensure reliability.
Practical Strategy for Comprehensive Order Tracking
To achieve comprehensive order tracking with IB API, you can combine different methods based on your needs:
- Use
reqOpenOrders()
for Specific Strategies: For single applications or strategies, usereqOpenOrders()
with a dedicated client ID to keep track of all programmatically placed orders. - Supplement with
reqAllOpenOrders()
: If you need to track manual orders or get an overview of all orders across clients, periodically usereqAllOpenOrders()
. - Real-Time Updates with
reqAutoOpenOrders(True)
: Use this with caution—rely onclientId=0
for continuous updates, but be prepared to handle missing updates and supplement with polling if needed.
Summary: Methods for Order Tracking
Method | What You Get | What You DON’T Get | Common Use |
---|---|---|---|
reqOpenOrders() | Orders placed by current client ID. | Does not capture manual or other client orders. | Common for single automated strategies. |
reqAllOpenOrders() | One-time snapshot of all open orders. | No updates for new orders after request. | Common when both manual and automated orders are used. |
reqAutoOpenOrders(True) | Continuous updates of all orders. | Can be unreliable for manual orders, requires clientId=0 . | Common for real-time monitoring. |
Conclusion
For most use cases, reqOpenOrders()
combined with a unique client ID is the recommended way to track orders, ensuring your application only deals with orders it has placed. This is the most straightforward and effective approach for automated trading systems. For scenarios requiring a comprehensive view, reqAllOpenOrders()
and reqAutoOpenOrders()
can be used but require careful consideration to handle their limitations. By combining these tools strategically, you can maintain robust and reliable order tracking for all your trading activities.